Part 1 — Intro to Machine Learning with TensorFlow simplified.
Here are some things you should know before diving deep into this lessons :
Learning by doing is my thing, so most of the terminologies of machine learning and tensorflow in this lesson will be demonstrated using examples. If machine learning complex mathematics is what you want, you won’t get them here, but you will at least get everything else and understand the mathematical process intuitively
For this lesson, you are required to have tensorflow, and python (numpy and matplotlib) installed. You can easily find resources online on how to get the installations done. Also, you should have jupyter notebook installed on your laptop preferably or any IDLE of your choice. In case the installation becomes so difficult, you can use google colab notebook direcly on the internet and use “!pip install package_name” to do all installation.
You should be at least familiar with basic python to get the most out of the codes in this article, but I will try my best explaining the steps.
Now let’s dive into actual business What’s Machine Learning? Machine Learning is an application of artificial intelligence (AI) that provides systems the ability to automatically learn and improve from experience without being explicitly programmed. I will explain this better working you through an example:
Suppose you have the below input and output values; when you input 0, you get an out 32, when you input 8, you get an output of 46.4 etc. The question now is: Can you figure out what the Output value for an input value 38 will be?
Input values and its output values
If your answer is 100.4, you are a rock-star, but how did you figure that out? By building a simple linear system. You can agree with me that at the end of the day, the linear system looks like y = 1.8*x +32 which leads to the popularly known Celsius to Fahrenheit conversion. In other words, the inputs show the temperature values in Celsius, while the outputs represent the corresponding temperature in degrees Fahrenheit
what you just did by figuring out the exact relationship between input and output values is exactly what machine learning does. i.e given sets of inputs with their corresponding outputs, machine learning will figure out the correct algorithm to convert the inputs to the outputs. When I say algorithm, I mean the relationship between the inputs and outputs. Think of it this way:
Traditional Software Development: The input and the algorithm is known, and you write a function to produce an output. i.e 1. input data, 2. apply logic to it (algorithm), 3. get the output. Let see an example, suppose you wanna write a computer program that convert degrees Celsius to Fahrenheit using the relation we derived above, with software development, this program can be easily implemented in any programming language by using a function. Here is an example in python:
Machine Learning: The input and the output are known, but you don’t know the algorithm that creates the output given the input. i.e 1. take pairs of input and output, 2. figure out the algorithm. Since we don’t know the algorithm, it means we can’t write a function. Here is an example for a machine learning function in python:
But to solve this problem using machine learning, we will need to introduce the concept of neural network into the above function. The machine learning uses a neural network to learn the relation between these inputs and outputs. Neural network concept will be thoroughly treated in the part 2 of this lesson.
You can think of neural networks as stack of layers consisting some predefined math and internal variables. The input value is fed to the neural network and follows through the stack of layers. The math and internal variables are then apply to the input, and the output is produced. In order for the neural network to learn the correct relationship between the inputs and the outputs, we have to train it. We train our neural network by repeatedly letting the network try to map the input to the output. By training, the neural network tunes the internal variables by experience until it learns to produce the output given the inputs.
Tuning the internal variables is perform over a thousand or millions of iteration that is not visible to us. Now let see how this can be done in code. Instead of writing a function for our Celsius to Fahrenheit conversion just like a typical software developer, we will use machine learning to train some set of data and see if our machine can predict the output correctly, given the input.
To make life easier, I always approach building machine learning model using 6 simple steps. I won’t be explaining this in details in later articles, so know what each step does now.
Import all essential dependencies:
First we input TensorFlow and other python dependencies needed to build our model. Here I will be using Jupyter notebook:
Setting up training data:
For simplification, we will be using numpy to create a simple training data, later, we will be importing data directly from tensorflow. we create two arrays celsius_a and fahrenheit_a that we can use to train our model.
Some Machine Learning terminology
Feature — The input(s) to our model. In this case, a single value — the degrees in Celsius.
Labels — The output our model predicts. In this case, a single value — the degrees in Fahrenheit.
Example — A pair of inputs/outputs used during training. In our case a pair of values from celsius_a and fahrenheit_a at a specific index, such as (22,72).
Creating the model and Assembling layers into the model:
We will be using the simplest possible model called Dense Network, since the problem is very straightforward. This network requires only single layer, with single neuron.
We’ll call the layer l0 and create it by instantiating tf.keras.layers.Dense with the following configuration:
input_shape=[1] — This specifies that the input to this layer is a single value. That is, the shape is a one-dimensional array with one member. Since this is the first (and only) layer, that input shape is the input shape of the entire model. The single value is a floating point number, representing degrees Celsius.
units=1 — This specifies the number of neurons in the layer. The number of neurons defines how many internal variables the layer has to try to learn how to solve the problem (more later). Since this is the final layer, it is also the size of the model's output — a single float value representing degrees Fahrenheit. (In a multi-layered network, the size and shape of the layer would need to match the input_shape of the next layer.) Once layers are defined, they need to be assembled into a model. The Sequential model definition takes a list of layers as an argument, specifying the calculation order from the input to the output.
Compiling and Training the model:
Before training, the model has to be compiled. When compiled for training, the model is given:
Loss function — A way of measuring how far off predictions are from the desired outcome.
Optimizer function — A way of adjusting internal values in order to reduce the loss.
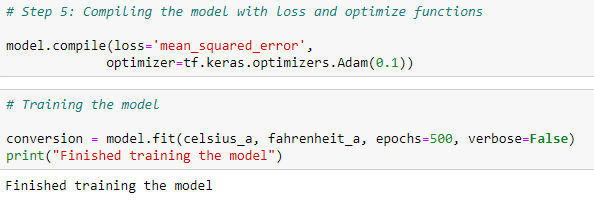
The optimizer and the loss function can be change pending on the need. This will be explain very well in the next article. One part of the Optimizer you may need to think about when building your own models is the learning rate (0.1 in the code above). This is the step size taken when adjusting values in the model. If the value is too small, it will take too many iterations to train the model. Too large, and accuracy goes down. Finding a good value often involves some trial and error, but the range is usually within 0.001 (default), and 0.1. The epochs argument specifies how many times this cycle should be run, and the verbose argument controls how much output the method produces. We call the fit method to train a model in machine learning
Visualizing loss magnitude
We visualize the loss magnitude so we can see how the loss of our model goes down after each training epoch. A high loss means that the Fahrenheit degrees the model predicts is far from the corresponding value in fahrenheit_a. Play around increasing the epoch number and learning rate to see how your model behaves.
Making Prediction to see how well our model performed
Now you have a model that has been trained to learn the relationship between celsius_a and fahrenheit_a. You can use the predict method to have it calculate the Fahrenheit degrees for a previously unknown Celsius degrees. Now let’s find the value of Fahrenheit degree if Celsius degree is 100. Using the equation we derived at the beginning of this lesson Fahrenheit(F) = 100 *1.8 +32 = 212. Now let see what our model predicts.
Now you just build a machine learning model that can convert Celsius degree to Fahrenheit degree with 99.5% accuracy (you can calculate this yourself using tensorflow or python mean squared error.) This kind of accuracy won’t happen when we are dealing with bigger problems, but the purpose of this is to learn the basics of machine learning.
Little note on Dense network: We used a dense layer to build our model above, but what is Dense layer? It is just a way of saying fully connected neural network layer. The neurons in each layer is connected to the neurons in the following layers.
The above single dense layer is exactly what we used to build our model. It takes x1 as the input, then iterate over and over to tune the internal variables w11 and b1 (weight (slope in general sense), and bias respectively) until they can predict with high accuracy.
You’ve learned the basic of machine learning and how to train a simple machine learning model. Next you will build a real life model using tensorflow mnist dataset. Watch out for part 2.